Python字符串与正则表达式详细介绍!
Python字符串与正则表达式详细介绍!
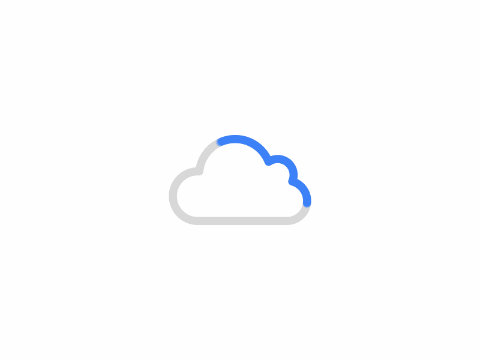
一、字符串相关操作
1.统计所输入字符串中单词的个数,单词之间用空格分隔。其运行效果如下图所示。
1
2
3
4
5
6
7
8
9
10
11
|
s = input('请输入字符串:') sum = 1 for i in s: if i == ' ': sum += 1 print('方法一:', end='') print('其中的单词总数有:', sum) list = s.split(' ') print('方法二:', end='') print('其中的单词总数有:', len(list)) |
2. 编写程序,给出一个字符串,将其中的字符“E”用空格替换后输出。
1
2
3
4
|
a = input ( '请输入一个字符串:' ) print ( '替换前:' ,a) a = a.replace( 'E' , ' ' ) print ( '替换后:' ,a) |
3. 从键盘交互式输人一个人的 18 位的身份证号,以类似于“2001 年 09 月 12 日”的形式输出该人的出生日期。
1
2
|
idc = input ( "请输入身份证号:" ) print ( str . format ( '出生日期:{0}年{1}月{2}日' ,idc[ 6 : 10 ],idc[ 10 : 12 ],idc[ 12 : 14 ])) |
4.将字符串'abcdefg'使用函数的方式进行倒序输出
1
2
|
list = 'abcdefg' print ( list [:: - 1 ]) |
5. 在我们的生活中,节假日的问候是必不可少的,请使用字符串格式化的方式写一个新年问候语模板.
1
2
|
name = input ( "请输入姓名:" ) print ( "祝{}新年快乐!" . format (name)) |
6. 用户输入一个字符串,将下标为偶数的字符提出来合并成一个新的字符串 A,再将下标为奇数的字符提出来合并成一个新的字符串 B,再将字符串 A 和 B 连接起来并输出。
1
2
3
4
5
6
|
s = input ( '请输入字符串:' ) A = s[ 0 :: 2 ] B = s[ 1 :: 2 ] print ( 'A=' ,A) print ( 'B=' ,B) print (A + B) |
7. 请根据下列需求,编写一个程序。用户输入一个字符串,请将字符串中的所有字母全部向后移动一位,最后一个字母放到字符开头,最后将新的字符串输出。
1
2
3
|
s = input ( '请输入字符串:' ) s_new = s[ - 1 ] + s[: len (s) - 1 ] #s[-1]表示s最后一位,s[:len(s)-1]表示切片到倒数第二位 print (s_new) |
8. 基于 input 函数,对输入的字符串进行处理,并将返回替换了某些字符的字符串,规则如下:
- 如果一个字母是大写辅音,请将该字符替换为“
Iron
”。 - 如果字母是小写辅音或非字母字符,则对该字符不执行任何操作。
- 如果一个字母是大写元音,请将该字符替换为“
Iron Yard
”。 - 如果一个字母是小写元音,请用“
Yard
”替换该字符。
1
2
3
4
5
6
7
8
9
10
|
import re text = input ( "请输入字符串:" ) for i in text: if i = = 'A' or i = = 'O' or i = = 'E' or i = = 'I' or i = = 'U' : a = re.sub( '[AOEIU]' , 'Iron Yard' ,text) if i = = 'a' or i = = 'o' or i = = 'e' or i = = 'i' or i = = 'u' : a = re.sub( '[aoeiu]' , 'Yard' ,text) if i > 'A' and i < 'Z' : a = re.sub( '[A-Z-[AOEIU]]' , 'Iron' ,text) print ( "替换后的字符为:" ,a) |
二、正则表达式相关操作
1. 写出能够匹配163 邮箱(@163.com)的正则表达式,并用 re.match 方法和邮箱 sda123(wer)u@163.com 作为测试验证。
1
2
3
4
5
6
|
import re s = input ( "请输入邮箱:" ) if re.match(r '.*?@163.com' ,s): print ( '是' ) else : print ( '不是' ) |
2. 利用 re 库中的 search、findall 或 search 函数从以下三个字符串中提取出所有的汉字,输出的结果分别为“大连理工大学”,“重庆大学”以及“中南财经大学” 。(提示:字符串 st2,str3 中有空格)。
- str1="""<td width="160">大连理工大学</td>"""
- str2="""<td width="160"><a href="../news/list_117.html"class="keyWord" target="_blank">重庆</a>大学</td>"""
- str3="""<td width="160"> 中南 <a href="../news/list_197.html"class="keyWord" target="_blank"> 财经 </a><ahref="../news/list_201.html" class="keyWord" target="_blank">政法</a>大学</td>"""
1
2
3
4
5
6
7
8
9
10
|
import re str1 = """<td width="160">大连理工大学</td>""" str2 = """<td width="160"><a href="../news/list_117.html" rel="external nofollow" rel="external nofollow" class="keyWord" target="_blank">重庆</a>大学</td>""" str3 = """<td width="160">中南<a href="../news/list_197.html" rel="external nofollow" rel="external nofollow" class="keyWord" target="_blank">财经</a><a href="../news/list_201.html" rel="external nofollow" rel="external nofollow" class="keyWord" target="_blank">政法</a>大学</td>""" re1 = re.search( """<td width="160">(.*?)</td>""" ,str1).group( 1 ) print (''.join( map ( str ,re1))) re2 = re.search( """<td width="160"><a href="../news/list_117.html" rel="external nofollow" rel="external nofollow" class="keyWord" target="_blank">(.*?)</a>(.*?)</td>""" ,str2).group( 1 , 2 ) print (''.join( map ( str ,re2))) re3 = re.search( """<td width="160">(.*?)<a href="../news/list_197.html" rel="external nofollow" rel="external nofollow" class="keyWord" target="_blank">(.*?)</a><a href="../news/list_201.html" rel="external nofollow" rel="external nofollow" class="keyWord" target="_blank">(.*?)</a>(.*?)</td>""" ,str3).group( 1 , 2 , 3 , 4 ) print (''.join( map ( str ,re3))) |
到此这篇关于Python字符串与正则表达式详细介绍的文章就介绍到这了。
学习资料见知识星球。
以上就是今天要分享的技巧,你学会了吗?若有什么问题,欢迎在下方留言。
快来试试吧,小琥 my21ke007。获取 1000个免费 Excel模板福利!
更多技巧, www.excelbook.cn
欢迎 加入 零售创新 知识星球,知识星球主要以数据分析、报告分享、数据工具讨论为主;
1、价值上万元的专业的PPT报告模板。
2、专业案例分析和解读笔记。
3、实用的Excel、Word、PPT技巧。
4、VIP讨论群,共享资源。
5、优惠的会员商品。
6、一次付费只需129元,即可下载本站文章涉及的文件和软件。